Adobe® Experience Manager (AEM) LiveCycle Connector enables seamless invocation of Adobe® LiveCycle® ES4 Document Services from within AEM web apps and workflows. LiveCycle provides a rich client SDK, which allows client applications to invoke LiveCycle services using Java™ APIs. AEM LiveCycle Connector simplifies using these APIs within the OSGi environment.
Once the package is installed, provide LiveCycle server details using Configuration settings in AEM Web Console.
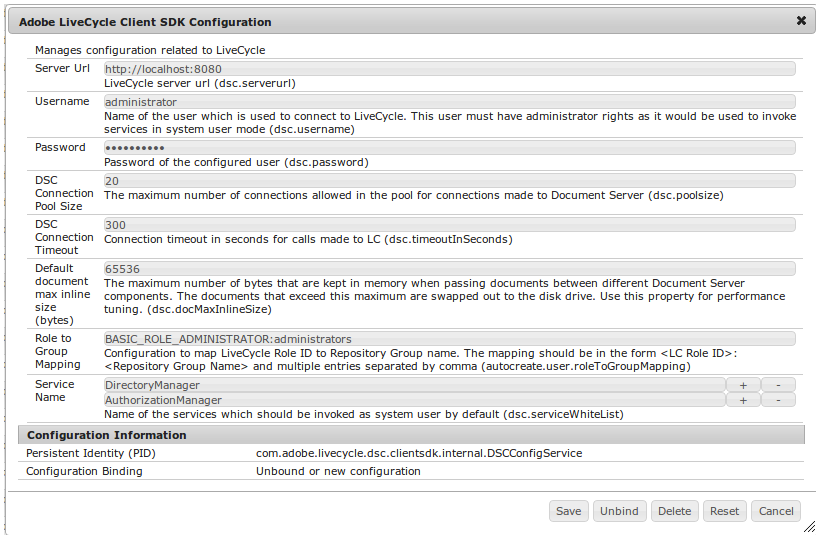
Although the properties are self explanatory, the important ones are as follows:
- Server URL - URL to the LiveCycle server. If you want LiveCycle and AEM to communicate over https, start AEM with the -Djavax.net.ssl.trustStore=<path to LC keystore> option.
- Username - Username of the account That will be used for making calls from AEM to LiveCycle. It can be any LiveCycle user who has the permissions to invoke Document Services.
- Password - User password.
- Service Name - Services that should be invoked using the user credentials provided in Username and Password fields. By default, no credentials are passed while invoking LiveCycle services.
Client applications can programmatically invoke LiveCycle services using a Java API, web services, Remoting, and REST. For Java clients, the application can make use of the LiveCycle SDK, which provides a Java API for invoking these services remotely. For example, to convert an Microsoft® Word Document to PDF, the client needs to invoke GeneratePDFService. The invocation flow consists of the following steps:
- Client instances as OSGi Service - The clients packaged as OSGI bundles are listed in the Document Services list section. Each client jar in turn registers the client instance as OSGi service with the OSGi Service Registry.
- User Credential Propagation - The connection details required to connect to LiveCycle server are now managed at a central place.
- ServiceClientFactory Service - Client application can still access the ServiceClientFactory instance if they need to invoke processes.
-
Add dependency to the required client jar in your maven pom.xml. At a minimum, you need to add dependency to adobe-livecycle-client and adobe-usermanager-client jars.
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-livecycle-client</artifactId> <version>11.0.0</version> </dependency> <dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-usermanager-client</artifactId> <version>11.0.0</version> </dependency> <dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-cq-integration-api</artifactId> <version>11.0.0</version> </dependency>
Now depending on the service to be invoked add the corresponding Maven dependency as per table below (Refer section Document Service List). For example for Generate PDF add following dependency
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-generatepdf-client</artifactId> <version>11.0.0</version> </dependency>
-
Get a handle to the service instance. If you are writing a Java class, you can use the Declarative Services annotations.
import com.adobe.livecycle.generatepdf.client.GeneratePdfServiceClient; import com.adobe.livecycle.generatepdf.client.CreatePDFResult; import com.adobe.idp.Document; @Reference GeneratePdfServiceClient generatePDF; ... Resource r = resourceResolver.getResource("/path/tp/docx"); Document sourceDoc = new Document(r.adaptTo(InputStream.class)); CreatePDFResult result = generatePDF.createPDF2( sourceDoc, extension, //inputFileExtension null, //fileTypeSettings null, //pdfSettings null, //securitySettings settingsDoc, //settingsDoc null //xmpDoc );
In the code snippet above, we invoke the createPDF API of GeneratePdfServiceClient to convert a document to PDF. It implements the same logic as explained in the quickstart example.
You can perform the same invocation in a JSP using the following code. The main difference here is the way we get access to GeneratePdfServiceClient using the Sling ScriptHelper
<%@ page import="com.adobe.livecycle.generatepdf.client.GeneratePdfServiceClient" %> <%@ page import="com.adobe.livecycle.generatepdf.client.CreatePDFResult" %> <%@ page import="com.adobe.idp.Document" %> GeneratePdfServiceClient generatePDF = sling.getService(GeneratePdfServiceClient.class); Document sourceDoc = ... CreatePDFResult result = generatePDF.createPDF2( sourceDoc, extension, //inputFileExtension null, //fileTypeSettings null, //pdfSettings null, //securitySettings settingsDoc, //settingsDoc null //xmpDoc );
Sample package
You can download and use the following sample package for converting a Microsoft Word document to PDF.
Download
Perform the following steps to use the sample package:
- Click aem-lc-connector-sample-pkg-1.0.0.zip file to download the sample package.
- Upload and install the attached package in AEM.
- To convert a Microsoft Word document to pdf, go to http://<hostname>:<port>/content/livecycle/connector/samples/convertdoc-toPDF.html.
- Choose the file to convert to PDF and click Convert.
- To browse through the code for this service using CRXDE Lite, navigate to apps/livecycle/connector/samples/pdfg/POST.jsp.
import com.adobe.livecycle.dsc.clientsdk.ServiceClientFactoryProvider; import com.adobe.idp.dsc.clientsdk.ServiceClientFactory; @Reference ServiceClientFactoryProvider scfProvider; ... ServiceClientFactory scf = scfProvider.getDefaultServiceClientFactory(); ...
Most of the Document Services in LiveCycle require authentication. It is possible to invoke these services without providing explicit credentials in the code. You can use any of the following options.
LiveCycle Client SDK configuration contains a setting about service names. This is a list of services for which the invocation logic uses administrator credential (part of same configuration) out of the box. For example, if you add DirectoryManager services (part of User Management API) to this list, any client code can directly use the service and the invocation layer automatically passes on the configured credentials as part of the request sent to LiveCycle server
As part of the integration, a new service RunAsManager is provided. It allows you to programmatically control credential to be used when making call to LiveCycle server.
import com.adobe.livecycle.dsc.clientsdk.security.PasswordCredential; import com.adobe.livecycle.dsc.clientsdk.security.PrivilegedAction; import com.adobe.livecycle.dsc.clientsdk.security.RunAsManager; import com.adobe.idp.dsc.registry.component.ComponentRegistry; @Reference private RunAsManager runAsManager; List<Component> components = runAsManager.doPrivileged(new PrivilegedAction<List<Component>>() { public List<Component> run() { return componentRegistry.getComponents(); } }); assertNotNull(components);
If you want to pass different credential, you can use the overloaded method that takes a PasswordCredential instance.
PasswordCredential credential = new PasswordCredential("administrator","password"); List<Component> components = runAsManager.doPrivileged(new PrivilegedAction<List<Component>>() { public List<Component> run() { return componentRegistry.getComponents(); } },credential);
If you are invoking a process or making direct use of ServiceClientFactory and creating an InvocationRequest, you can specify a property to indicate that invocation layer should use configured credentials.
import com.adobe.idp.dsc.InvocationResponse import com.adobe.idp.dsc.InvocationRequest import com.adobe.livecycle.dsc.clientsdk.ServiceClientFactoryProvider import com.adobe.idp.dsc.clientsdk.ServiceClientFactory import com.adobe.livecycle.dsc.clientsdk.InvocationProperties ServiceClientFactoryProvider scfp = sling.getService(ServiceClientFactoryProvider.class) ServiceClientFactory serviceClientFactory = scfp.getDefaultServiceClientFactory() InvocationRequest ir = serviceClientFactory.createInvocationRequest("sample/LetterSubmissionProcess", "invoke", new HashMap(), true); //Here we are invoking the request with system user ir.setProperty(InvocationProperties.INVOKER_TYPE,InvocationProperties.INVOKER_TYPE_SYSTEM) InvocationResponse response = serviceClientFactory.getServiceClient().invoke(ir);
Document Services list
Adobe LiveCycle Client SDK API bundle
Adobe LiveCycle Client SDK Bundle
Adobe LiveCycle TaskManager Client bundle
Adobe LiveCycle Workflow Client Bundle
Adobe LiveCycle PDF Generator Client bundle
Adobe LiveCycle Application Manager Client bundle
Adobe LiveCycle Assembler Client bundle
Adobe LiveCycle Form Data Integration Client bundle
Adobe LiveCycle Forms Client bundle
Adobe LiveCycle Output Client bundle
Adobe LiveCycle Reader Extensions Client bundle
Adobe LiveCycle Rights Manager Client bundle
Adobe LiveCycle Signatures Client bundle
Services exposed:
- com.adobe.idp.um.api.AuthenticationManager
- com.adobe.idp.um.api.DirectoryManager
- com.adobe.idp.um.api.AuthorizationManager
- com.adobe.idp.dsc.registry.service.ServiceRegistry
- com.adobe.idp.dsc.registry.component.ComponentRegistry
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-livecycle-client</artifactId> <version>11.0.0</version> </dependency> <dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-usermanager-client</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.livecycle.dsc.clientsdk.security.RunAsManager
- com.adobe.livecycle.dsc.clientsdk.ServiceClientFactoryProvider
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-livecycle-cq-integration-api</artifactId> <version>1.1.10</version> </dependency>
Services exposed:
- com.adobe.idp.taskmanager.dsc.client.task.TaskManager
- com.adobe.idp.taskmanager.dsc.client.TaskManagerQueryService
- com.adobe.idp.taskmanager.dsc.client.queuemanager.QueueManager
- com.adobe.idp.taskmanager.dsc.client.emailsettings.EmailSettingService
- com.adobe.idp.taskmanager.dsc.client.endpoint.TaskManagerEndpointClient
- com.adobe.idp.taskmanager.dsc.client.userlist.UserlistService
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-taskmanager-client</artifactId> <version>11.0.0</version> </dependency>
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-workflow-client-sdk</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.livecycle.generatepdf.client.GeneratePdfServiceClient
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-generatepdf-client</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.idp.applicationmanager.service.ApplicationManager
- com.adobe.livecycle.applicationmanager.client.ApplicationManager
- com.adobe.livecycle.design.service.DesigntimeService
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-applicationmanager-client-sdk</artifactId> <version>11.0.0</version> </dependency>
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-assembler-client</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.livecycle.formdataintegration.client.FormDataIntegrationClient
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-formdataintegration-client</artifactId> <version>11.0.0</version> </dependency>
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-forms-client</artifactId> <version>11.0.0</version> </dependency>
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-output-client</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.livecycle.readerextensions.client.ReaderExtensionsServiceClient
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-reader-extensions-client</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.livecycle.rightsmanagement.client.DocumentManager
- com.adobe.livecycle.rightsmanagement.client.EventManager
- com.adobe.livecycle.rightsmanagement.client.ExternalUserManager
- com.adobe.livecycle.rightsmanagement.client.LicenseManager
- com.adobe.livecycle.rightsmanagement.client.WatermarkManager
- com.adobe.livecycle.rightsmanagement.client.PolicyManager
- com.adobe.livecycle.rightsmanagement.client.AbstractPolicyManager
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-rightsmanagement-client</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.livecycle.signatures.client.SignatureServiceClientInterface
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-signatures-client</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.truststore.dsc.TrustConfigurationService
- com.adobe.truststore.dsc.CRLService
- com.adobe.truststore.dsc.CredentialService
- com.adobe.truststore.dsc.CertificateService
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-truststore-client</artifactId> <version>11.0.0</version> </dependency>
Services exposed:
- com.adobe.repository.bindings.ResourceRepository
- com.adobe.repository.bindings.ResourceSynchronizer
Maven dependencies:
<dependency> <groupId>com.adobe.livecycle</groupId> <artifactId>adobe-repository-client</artifactId> <version>11.0.0</version> </dependency>